Setting Up the Project
This guide provides step-by-step instructions for setting up your ASP.NET Core web application, "YourSite," to integrate with K12NET's APIs. By following these steps, you will prepare your project environment, configure necessary dependencies, and establish the foundation for implementing Single Sign-On (SSO) and API communication.
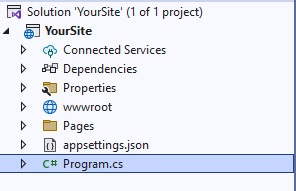
Prerequisites
- .NET Core SDK (version specified by your project requirements)
- Visual Studio or Visual Studio Code
- A K12NET developer account and access credentials (client_id, client_secret)
Step 1: Create Your ASP.NET Core Project
- Open Visual Studio or Visual Studio Code.
- Create a new project and select "ASP.NET Core Web Application."
- Choose the appropriate project template (e.g., MVC or Razor Pages) based on your preference.
- Name your project "YourSite" and select a location for your project files.
Step 2: Configure Authentication
To integrate K12NET's SSO, configure the authentication services in your project.
-
In the
Program.cs
file, add the following service :builder.Services.AddAuthentication(opt => opt.DefaultScheme = CookieAuthenticationDefaults.AuthenticationScheme).AddCookie();
-
Program.cs content should be similar to below:
using Microsoft.AspNetCore.Authentication.Cookies; namespace YourSite { public class Program { public static void Main(string[] args) { var builder = WebApplication.CreateBuilder(args); builder.Services.AddRazorPages(); builder.Services.AddAuthentication(opt => opt.DefaultScheme = CookieAuthenticationDefaults.AuthenticationScheme).AddCookie(); var app = builder.Build(); if (!app.Environment.IsDevelopment()) { app.UseHsts(); } app.UseStaticFiles(); app.UseRouting(); app.UseAuthentication(); app.UseAuthorization(); app.MapRazorPages(); app.Run(); } } }
Step 3: Configure API Access Paremeters
Ensure your application has the K12NET Partner information.
-
In the
appsettings.json
file, add theK12NETPartnerInfo
element:"K12NETPartnerInfo": { "url": "https://api-test.k12net.com", "client_id": "YOUR_CLIENT_ID", "client_secret": "YOUR_CLIENT_SECRET", "redirect_uri": "https://localhost:5178/Signin-K12NET" }
- Make sure you change the redirect_uri value according to your application's port.
Step 4: Build and Run
- Build your project to ensure all dependencies are correctly installed and configured.
- Run your project to verify that the initial setup is complete and functional.
Conclusion
You have now successfully set up your ASP.NET Core project, "YourSite," with the foundational elements for integrating with K12NET's APIs and implementing SSO. The next steps involve diving deeper into the specific functionalities you wish to incorporate, such as user authentication, data retrieval, and more, which will be covered in subsequent guides.